Now we need a tool to store the value of our counted collectables. And another tool to add to that value as we collect and count them. Let's add this tool to our PlayerController script. Select the Player game object and open the PlayerController script for editing.
Let's add a private variable to hold our count of collectables we've picked up. This will be an int, as our count will be a whole number we won't be collecting partial objects. And let's call it Count. Type private int count; So in our game we will first start with a count of 0.
Then we will need to increment our count value by 1 when we pick up a new object. We need to set our count value to 0. As this variable is private we don't have any access to it in the inspector. This variable is only available for use within this script.
And as such we will need to set it's starting value here in the script. There are several ways we can set the starting value of count but in this assignment we will do it in the Start function. In Start set our count to be equal to 0. Type count = 0 Next we need to add to count when we pick up our collectable game objects.
We will pick up our objects in onTriggerEnter2D. If the other game object has the tag Pickup. So this is where we add our counting code. After setting the other game object's active state to false we'll set our new value for count to be equal to our old value plus 1.
We'll type count = count + 1. There are other ways to add, count up or increment a value when coding in Unity, but this one is very easy to understand and this is the one that we're going to use in this assignment. Let's save our script and return to Unity. Now we can store and increment our count but we have no way of displaying it.
It would also be good to display a message when the game is over. To display text we will be using Unity's user interface, or UI toolset. First let's create a new UI text element from the hierarchy's Create menu. We seem to have gotten more than we've bargained for.
We don't just have a UI text element but we've also created a parent canvas element and an EventSystem game object. These are all required by the UI toolset. The single most important thing about these additional items is that all UI elements must be the child of a canvas to behave correctly. For more information on the UI tools including the canvas and the EventSystem please see the information linked below.
Let's rename the text element CountText. With the CountText still highlighted place the cursor over the scene view and press the F key to frame select it. Let's zoom out so that we can see where the text is positioned relative to the entire canvas. If for some reason this doesn't appear in the centre then we can use the context sensitive gear menu to reset the rect transform.
Now that our text is centred let's customise this element a bit. The default text is a bit dark, let's make the text colour yellow so it's easier to see. Click on the colour swatch in the colour picker let's set the red value to 255, the green value to 255 and the blue value to 0. You can do this by clicking and dragging or entering the values numerically.
Close the colour picker. Now let's add some placeholder text and replace the string New Text with Count Text. Currently the text element is in the centre of the screen because it's anchored to the centre of it's parent, which is in this case the canvas. If is worth noting that the transform component on UI elements is different from that of other game objects in Unity.
For UI elements the standard transform has been replaced with the rect transform, which takes in to account many specialised features necessary for a versatile UI system including anchoring and positioning. For more information on the rect transform please see the information linked below. One of the easiest ways to move the CountText element in to the upper left is to anchor it to the upper left corner of the canvas rather than to it's centre. When we re-anchor this text element we also want to set the pivot and the position based on the new anchor.
To do this open the Anchors And Presets menu by clicking on the button displaying the current anchor preset. Hold down the Shift and Option keys on Mac, or shift and Alt keys on Windows and select the upper left preset by clicking on it. That's done it. Now it looks budged up against the corner of the game view.
Let's give it some space between the text and the edges of the screen. As we're anchored to the upper left corner of the canvas and we've set our pivot to the upper left corner as well. The easiest way to give the text a little breathing room is to change the rect transform's position X and position Y values. In this case an X position of 10 and a Y position of -10 seem about right.
This gives us some room around it yet it's still up and out of the way. Now let's wire up the UI text element to display our count value. Let's start by opening the PlayerController script for editing. Before we can code anything related to any UI elements we need to tell our script more about them.
The details about the UI. Toolset are held in what's called a namespace. We need to use this namespace just as we are using UnityEngine and System.Collections. So to do this, at the top of our script we'll write using UnityEngine.UI.
With this in place we can now write our code. First create a new public text variable called CountText to hold a reference to the UI Text component on our UI Text game object. Type public Text countText We need to set the starting value of the UI text's Text property. WE can do this in Start as well.
Write countText.Text = "Count: " + count.ToString () And we need the parenthesis. Now this line of code must be written after the line setting our count value because Count must have some value for us to set the text with. Now we also need to update this text property every time we pick up a new collectable, so in OnTriggerEnter after we increment our count value let's write again countText.Text = "Count: " + count.ToString () We've now written the same line of code twice in the same script. This is generally bad form.
One way to made this a little more elegant is to create a function that does the work in one place and we simply call this function every time we need it. Let's create a new function called SetCountText. Type void SetCountText followed by an empty set of parenthesis and an empty set of brackets. Now let's move one instance of this line of code in to the function by cutting and pasting it.
And in it's place let's put a line of code simply calling the function. Finally let's replace the other line with the function call as well. Save and return to Unity. Now we see our PlayerController script has a new text property.
We can associate a reference to our Count Text simply by dragging and dropping the CountText game object on to this slot. Unity will find the text component on the game object and correctly associate the reference. Let's save the scene and play. Great, now not only do we collect these objects but we can count them now.
Let's exit play mode. We need to display a message when we have collected all of the pickups. To do this we will need another UI text object. Again, using the hierarchy's Create menu make a new UI text game object.
Rename it WinText. Note how the new UI text element is automatically added to our canvas. We want this text to display in the centre of the game space but up a little bit so it doesn't cover the Player game object. To do this let's adjust the rect transform's position Y element as by default this UI text element is anchored to the centre of the canvas.
A value of about 75 feels good. Let's also adjust the paragraph alignment in the text component. Next let's adjust the colour and set it to the same yellow we used for our previous text element. Let's make the text a little larger by setting the font size to 24.
Finally let's add some place holder text and replace the string New Text with Win Text. Let's save the scene and swap back to our scripting editor. We need to add a reference for this UI text element. Let's create a new public text variable and call it WinText.
Type public Text winText. Now let's set the starting value for WinText's text property. This is set to an empty string or two double quote marks with no content. This text property will start empty then in the SetCountText function let's write if count is greater than or equal to 12, which is the total number of objects we have in the game to collect, then our winText.Text equals You Win! Type if count is greater than or equal to 12 winText.Text = YouWin! Let's save this script and return to Unity.
Again on our Player our player controller has a new UI text property. We can associate the component again by dragging the WinText game object in to the new slot. Let's save the scene and play. So we're picking up our game objects, we're counting our collectables and we win! And as we can see when we have collected 12 objects we display the You Win! Text.
In the next and last assignment of this series we will build the game and deploy it using a stand alone player. Subtitles by the Amara.Org community.
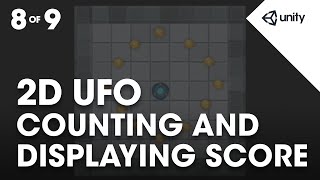
No comments:
Post a Comment